How To Use Python Sleep – Add Delay to Python Script
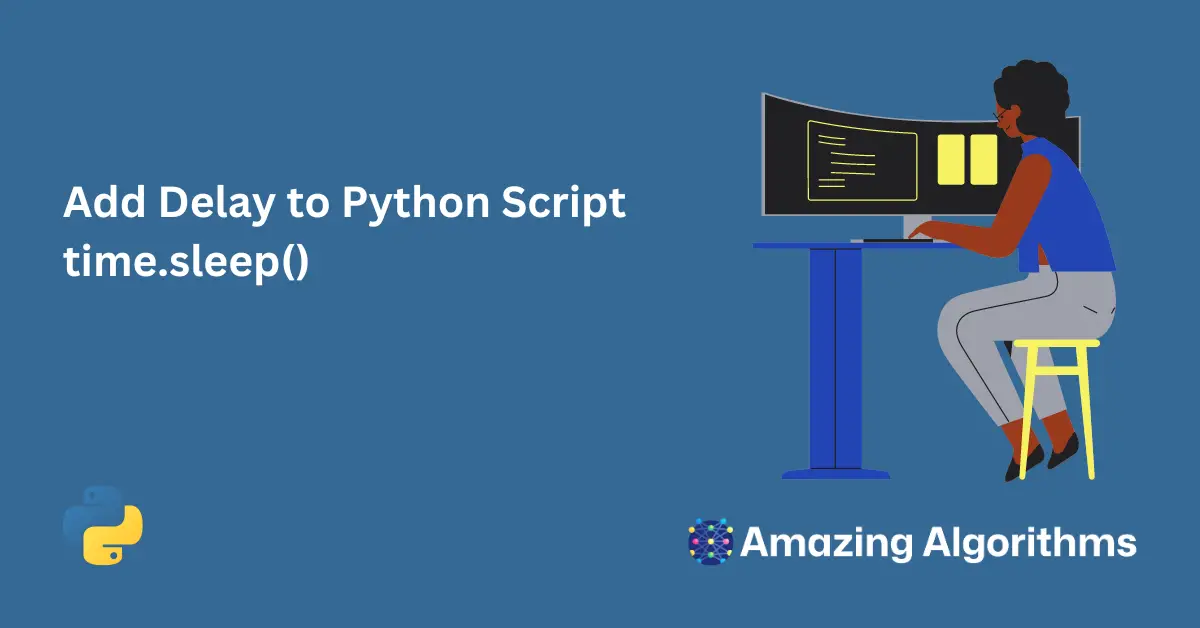
Delving into the realms of Python programming reveals the versatility and power of its libraries and functions. Among these, the sleep function stands out as a fundamental tool for managing time delays in your code. This function, residing within Python’s time module, offers a straightforward yet crucial capability – pausing the execution of a program for a specified duration.
Why Use Python’s Sleep Function?
The utility of the sleep function extends across various applications, from pacing the execution of tasks to synchronizing processes in both simple scripts and complex systems. It proves especially valuable in:
- Simulating delays: Whether you’re testing timeouts or mimicking real-world timings, sleep allows you to introduce precise pauses in your program’s execution.
- Throttling API calls: When interacting with web services, respecting rate limits is crucial. The sleep function helps manage request intervals to avoid exceeding these limits.
- Creating animations: In graphical applications or console-based animations, controlled delays between frames are essential for achieving the desired visual effect.
Implementing Sleep in Your Python Code
Integrating the sleep function into your Python scripts is a straightforward process, involving a simple import from the time module and a call to sleep() with the desired delay in seconds as its argument. The following snippet illustrates its basic usage:
import time
print("This message is displayed immediately.")
time.sleep(5) # Pauses execution for 5 seconds
print("This message is displayed after a 5-second delay.")
This simplicity belies the function’s adaptability, allowing it to fit seamlessly into a wide array of programming scenarios, from beginner-level scripts to advanced applications.
As we continue to explore the sleep function in Python, we’ll delve deeper into its applications, best practices, and nuances that make it an indispensable tool in your programming toolkit. Stay tuned for more insights in the upcoming sections of this article.
Advanced Usage and Considerations
While the sleep function is straightforward in its basic form, understanding its nuances can enhance its utility in more complex scenarios. Here are some advanced considerations:
- Integration with Threading: In multithreaded applications, sleep can be used to manage the execution flow of different threads, allowing for timed coordination without blocking the entire program’s execution.
- Handling Interrupts: When using sleep in scripts that may need to respond to interrupts, such as keyboard interrupts or signals, it’s essential to design your code to handle such exceptions gracefully.
- Subsecond Delays: The sleep function supports floating-point numbers as arguments, enabling precise subsecond delays for scenarios requiring high-resolution timing.
Practical Applications of Sleep
The sleep function finds its place in a multitude of practical applications, demonstrating its versatility. A few examples include:
- Automated Testing: In automated tests, especially those involving UI or network operations, introducing delays can ensure that elements are loaded or responses are received before assertions are made.
- Web Scraping: To prevent overwhelming a server with rapid, consecutive requests, adding delays between each request can promote respectful scraping practices.
- Game Development: For turn-based games or time-based events, sleep can regulate the pace, ensuring gameplay unfolds at a controlled speed.
Best Practices for Using Sleep
While sleep is invaluable, its misuse can lead to inefficiencies or unintended behavior in your applications. Here are some best practices:
- Avoid Excessive Use in GUI Applications: In graphical applications, overusing sleep can make the interface unresponsive. Consider event-driven programming or timers for better user experiences.
- Be Mindful of Sleep in Loops: When using sleep within loops, especially in networked applications, ensure that the cumulative delay does not detrimentally affect performance or user experience.
- Combine with Proper Error Handling: Especially in scripts that may run unattended, ensure that your use of sleep is paired with robust error handling to cope with unexpected interruptions or conditions.
As we delve further into the world of Python programming, the sleep function remains a fundamental tool, its simplicity belying the depth of control it offers over the temporal aspects of code execution. In the final section, we’ll explore additional insights and tips to maximize the effectiveness of sleep in your Python projects.
Optimizing Sleep in Complex Projects
In larger or more complex Python projects, the sleep function’s role becomes more nuanced but equally critical. Here are some tips for optimizing its use:
- Event-Driven Sleeping: In applications where responsiveness is key, consider using event-driven mechanisms that can wake up your program based on specific triggers rather than relying solely on fixed sleep intervals.
- Adaptive Sleeping: In scenarios where waiting times can vary (such as polling for a resource), implementing adaptive sleeping strategies can improve efficiency by dynamically adjusting wait times based on past experience or current conditions.
- Combining with Concurrency: For I/O-bound tasks, combining sleep with asynchronous programming or threading can allow your application to perform other operations while waiting, thereby enhancing overall performance.
Common Pitfalls and How to Avoid Them
Despite its simplicity, incorrect usage of sleep can lead to common pitfalls. Awareness and strategic planning can help avoid these:
- Sleeping in Critical Sections: Avoid placing sleep calls within critical sections of code where time sensitivity is crucial. This can lead to bottlenecks and performance degradation.
- Over-reliance on Sleep for Synchronization: Using sleep as a primary method for thread or process synchronization can lead to unreliable behavior. Explore Python’s threading or multiprocessing modules for more robust solutions.
- Ignoring System Clock Adjustments: Be mindful that system clock adjustments or daylight saving time changes can affect sleep durations. Consider using monotonic clocks if absolute precision is necessary.
Leveraging Sleep in Creative Ways
While typically used for adding delays, the sleep function can be creatively adapted for various other purposes, such as:
- Simulating Workloads: In performance testing or simulations, sleep can represent processing time, allowing you to model and analyze system behavior under different load conditions.
- Rate Limiting: In applications interacting with APIs or external services, sleep can help enforce rate limits, ensuring your application stays within usage policies and avoids throttling.
- Energy Conservation: In battery-powered or energy-sensitive applications, judicious use of sleep can conserve energy by reducing CPU usage during idle periods.
In conclusion, while sleep is a simple function at its core, its proper use requires thoughtful consideration. By understanding its behavior, integrating it wisely into your projects, and avoiding common pitfalls, you can harness the power of controlled pauses to create more efficient, responsive, and user-friendly Python applications.