How do I reverse a string in Python?
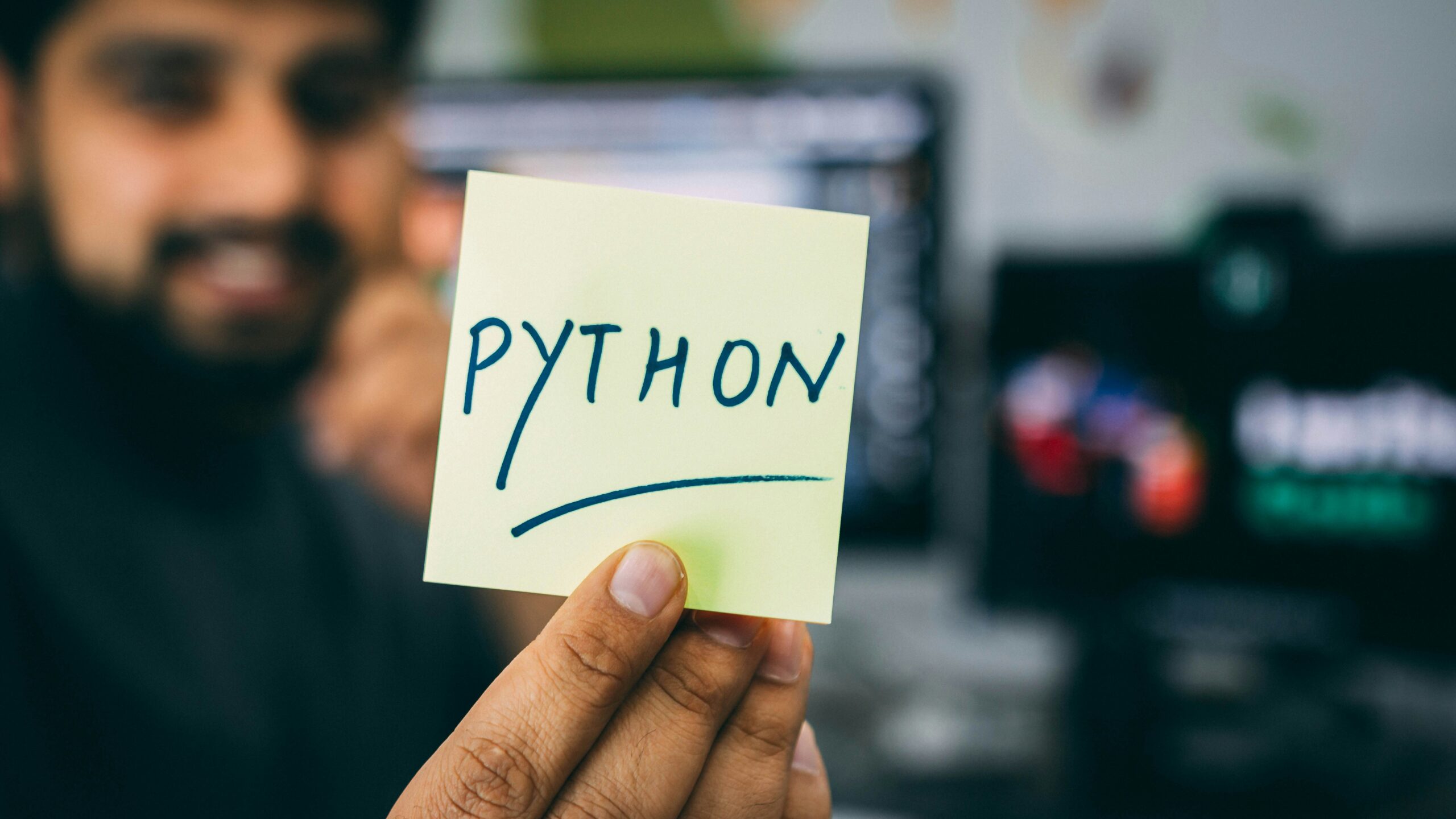
Python is a versatile and powerful programming language that offers a wide range of functionalities. One common task that you may encounter when working with strings in Python is reversing them. In this article, we will explore different methods to reverse a string in Python.
Method 1: Using String Slicing
One straightforward way to reverse a string in Python is by using string slicing. String slicing allows you to extract a portion of a string by specifying the start and end indices.
Here’s an example:
string = "Hello, World!"
reversed_string = string[::-1]
print(reversed_string)
The output of this code will be:
!dlroW ,olleH
By specifying a step value of -1 in the slicing operation ([::-1]
), we are effectively reversing the string.
Method 2: Using the reversed() Function
Python provides a built-in function called reversed()
that can be used to reverse an iterable, including strings. This function returns a reverse iterator, which can be converted back into a string using the join()
method.
Here’s an example:
string = "Hello, World!"
reversed_string = ''.join(reversed(string))
print(reversed_string)
The output of this code will be the same as the previous example:
!dlroW ,olleH
Using the reversed()
function provides a more concise way to reverse a string, especially if you need to perform additional operations on the reversed string.
Method 3: Using a For Loop
Another approach to reverse a string in Python is by using a for loop. This method involves iterating over the characters in the string from the last index to the first index and appending them to a new string.
Here’s an example:
string = "Hello, World!"
reversed_string = ""
for char in string:
reversed_string = char + reversed_string
print(reversed_string)
The output of this code will be the same as the previous examples:
!dlroW ,olleH
This method allows for more flexibility, as you can perform additional operations on each character while reversing the string.
Method 4: Using the join() Method
Python’s join()
method can also be used to reverse a string. This method joins all elements of an iterable into a single string, using a specified separator.
Here’s an example:
string = "Hello, World!"
reversed_string = ''.join(string[i] for i in range(len(string)-1, -1, -1))
print(reversed_string)
The output of this code will be the same as the previous examples:
!dlroW ,olleH
Using the join()
method in this way allows you to reverse a string without the need for additional variables or loops.
Conclusion
In this article, we explored four different methods to reverse a string in Python. Whether you prefer using string slicing, the reversed()
function, a for loop, or the join()
method, you now have multiple options to achieve the desired result.
Remember to choose the method that best suits your specific needs and coding style. Experiment with these approaches and perhaps even combine them in creative ways to tackle more complex string manipulation tasks. Each method has its own advantages and trade-offs in terms of readability, efficiency, and suitability for certain scenarios.
Using string slicing is by far the simplest and most Pythonic way to reverse a string. It’s concise, easy to read, and typically performs well for most use cases. However, it’s worth noting that this method creates a new string, which might not be the most memory-efficient approach for very large strings.
The reversed()
function, coupled with the join()
method, offers a more explicit way to reverse a string, which can enhance readability for those unfamiliar with Python’s slicing syntax. This method is also useful when dealing with more complex data structures or when you need to reverse elements in a way that slicing cannot accommodate.
Utilizing a for loop to reverse a string gives you the most control over the process. This method can be advantageous in scenarios where you need to perform additional operations on each character during the reversal process. However, this approach can be more verbose and less efficient compared to other methods, so it’s typically used when you have specific, unique requirements.
Lastly, the join()
method with a generator expression is a powerful combination that can be used for reversing strings and much more. This approach is both flexible and efficient, especially when dealing with large data sets or when you need to integrate the string reversal into a more complex data processing pipeline.
In conclusion, Python offers multiple ways to reverse a string, each with its own set of pros and cons. Whether you’re working on a simple script or a complex application, understanding these different methods allows you to write more efficient, readable, and Pythonic code. As always, the best approach depends on your specific project requirements, coding style, and performance considerations. Experiment with these methods, understand their nuances, and you’ll be well-equipped to handle a wide range of string manipulation tasks in Python.